Flux with Python: Up and running in minutes
Last month, I was stuck trying to generate custom images for a client project. After hours of frustration with YouTube tutorials and clunky UI tools, I discovered how I can use python code and Flux to create images.
Flux is a powerful image generation model that works seamlessly with Python. It lets you create amazing images right from your code. No need to fiddle with GUIs or complex APIs!
In this guide, I'll show you how you can use Flux with Python with a small open-source project I created. The project is tweaked to run on Apple Silicon, but can easily be updated to run on Cuda if necessary. Let's get started!
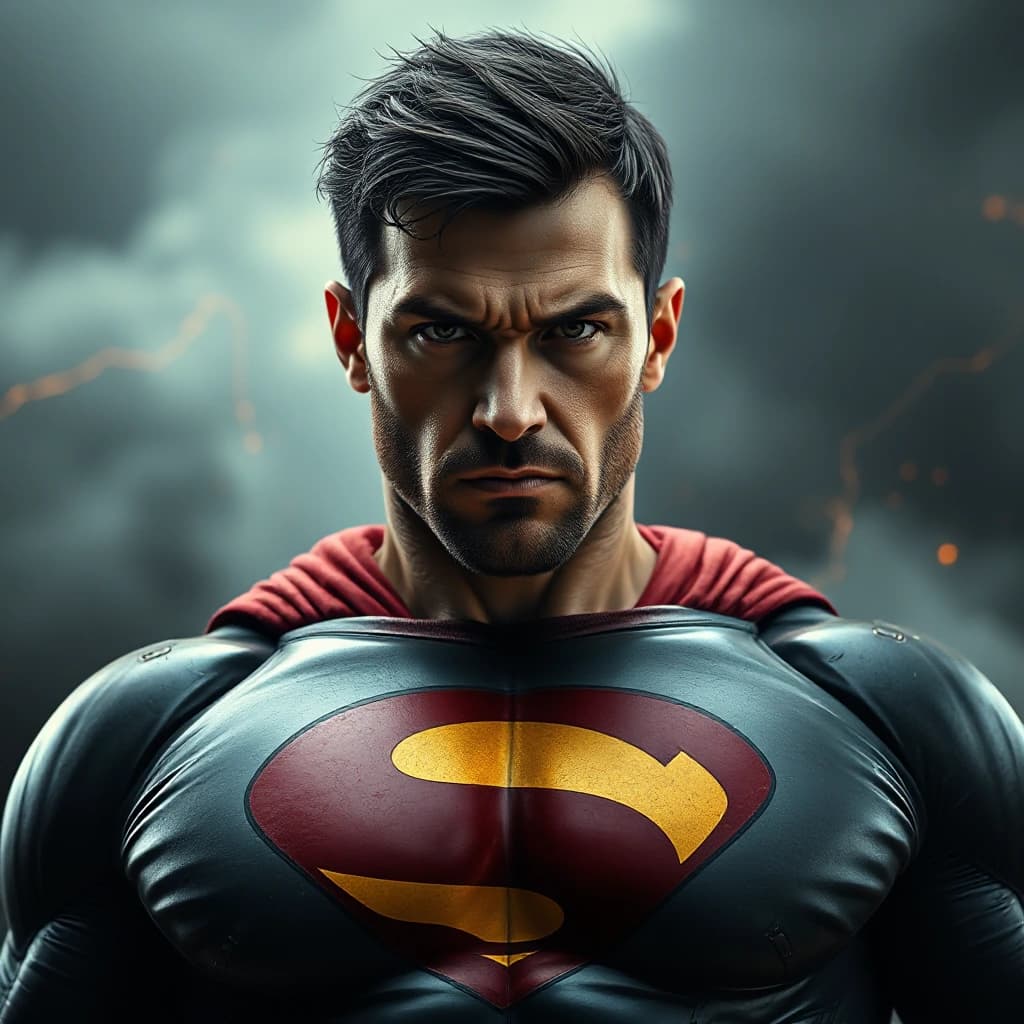
Setting Up Flux
First, let's get Flux ready to use:
- Get the code:
git clone https://github.com/einar-hansen/flux-python-package.git
cd flux-python-package
- Create a virtual environment:
python3 -m venv flux_env
source flux_env/bin/activate
- Install what you need:
pip install git+https://github.com/huggingface/diffusers.git
pip install transformers torch pillow pyyaml term-image accelerate protobuf sentencepiece
Note! The only way I could make diffusers
work was to use diffusers
directly from GitHub. The pip version gave the error ImportError: cannot import name 'FluxImg2ImgPipeline' from 'diffusers'
.
Your First Flux Image
Now, let's create an image:
python run_flux.py --model schnell --mode text2img "A city at night with neon lights"
This tells Flux to use the Schnell model to create an image based on our description. Your new image will be in the images
folder. On my MacBook it takes about 1 minute to create a new image. So have a little patience.
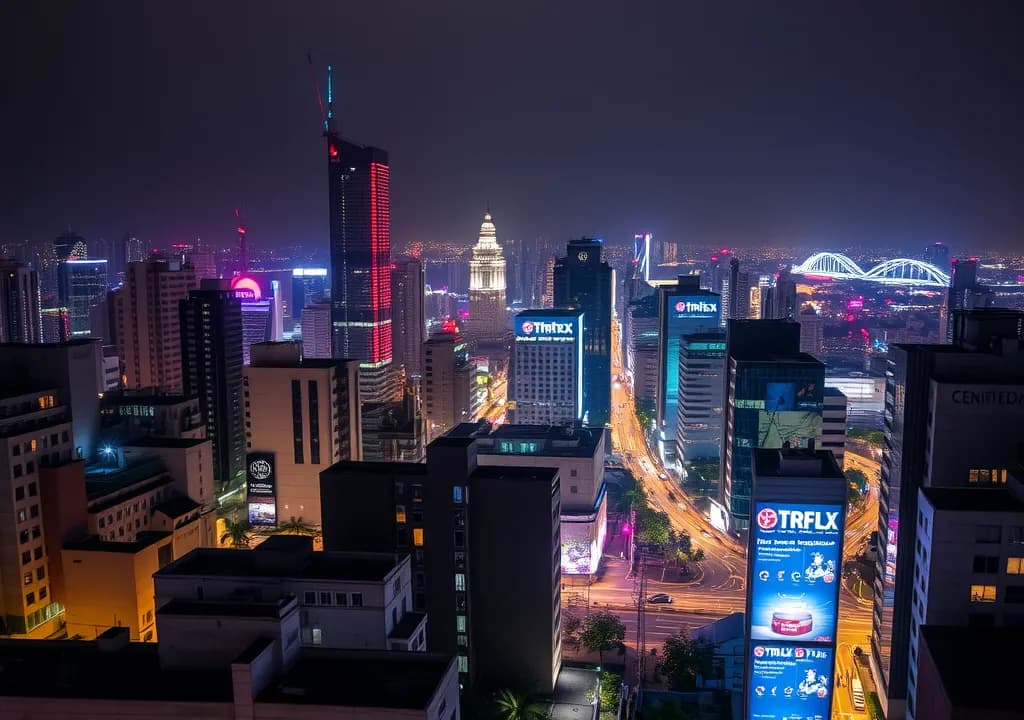
An example of what Flux might generate from our prompt.
Advanced Flux Features
Changing Existing Images
Flux can also change images you already have, with dev
:
python run_flux.py --model dev --mode img2img --strength 0.85 \
--num_inference_steps 50 --input_image images/city.png \
"turn the city into a jungle"
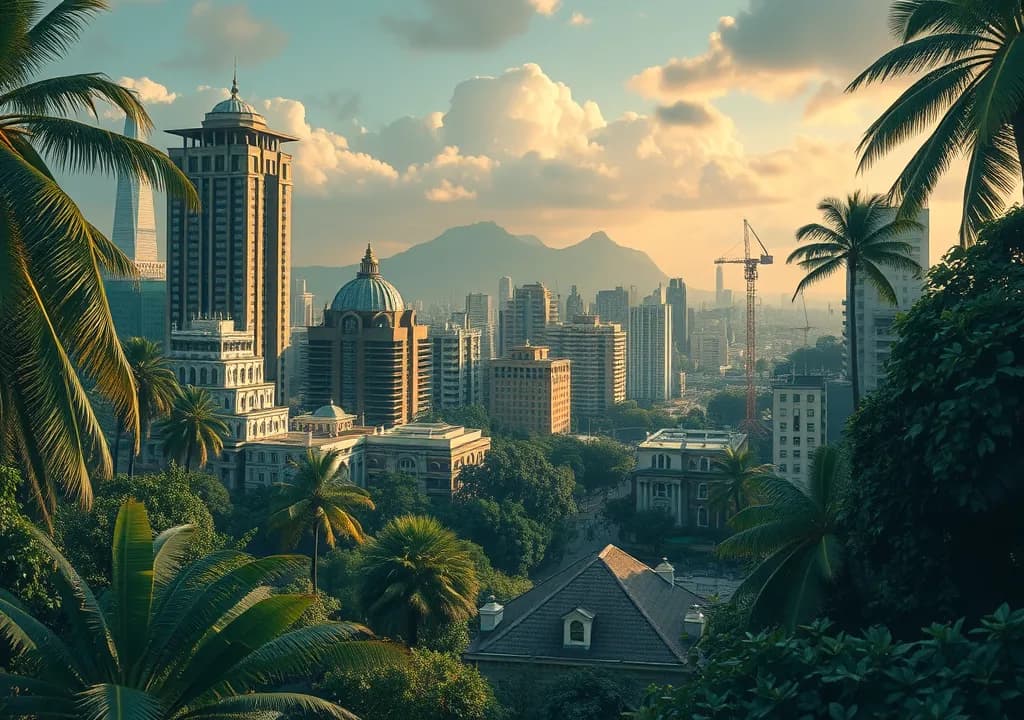
And with schnell
:
python run_flux.py --model schnell --mode img2img --strength 0.80 \
--num_inference_steps 5 --input_image images/city.png \
"turn the city into a jungle"
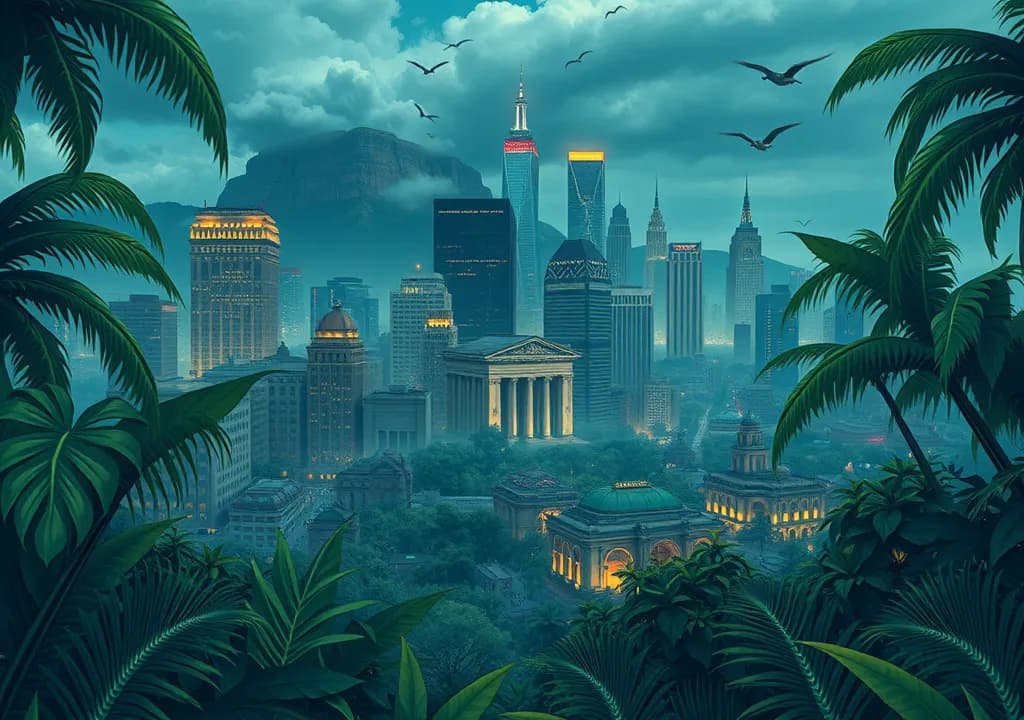
This takes your city image and adds a jungle to it. The strength
(from 0 to 1) controls how much it changes.
Making Images Bigger
Need a bigger image? Flux can do that too:
python run_flux.py --model dev --mode upscale \
--input_image images/small_portrait.png \
"make it clearer and bigger"
This only works with the Dev model. It's slower but gives great results. I often use this for client work when they need high-res images.
Customizing Flux
Flux lets you tweak many settings:
--guidance_scale
: How closely it follows your description--num_inference_steps
: More steps mean better quality but take longer--width
and--height
: Image size--randomness
: Adds variety to your images
Here's an example:
python run_flux.py --model schnell --mode text2img --guidance_scale 7.5 \
--num_inference_steps 10 --width 1024 --height 1024 --randomness \
"A flying car over a futuristic city"
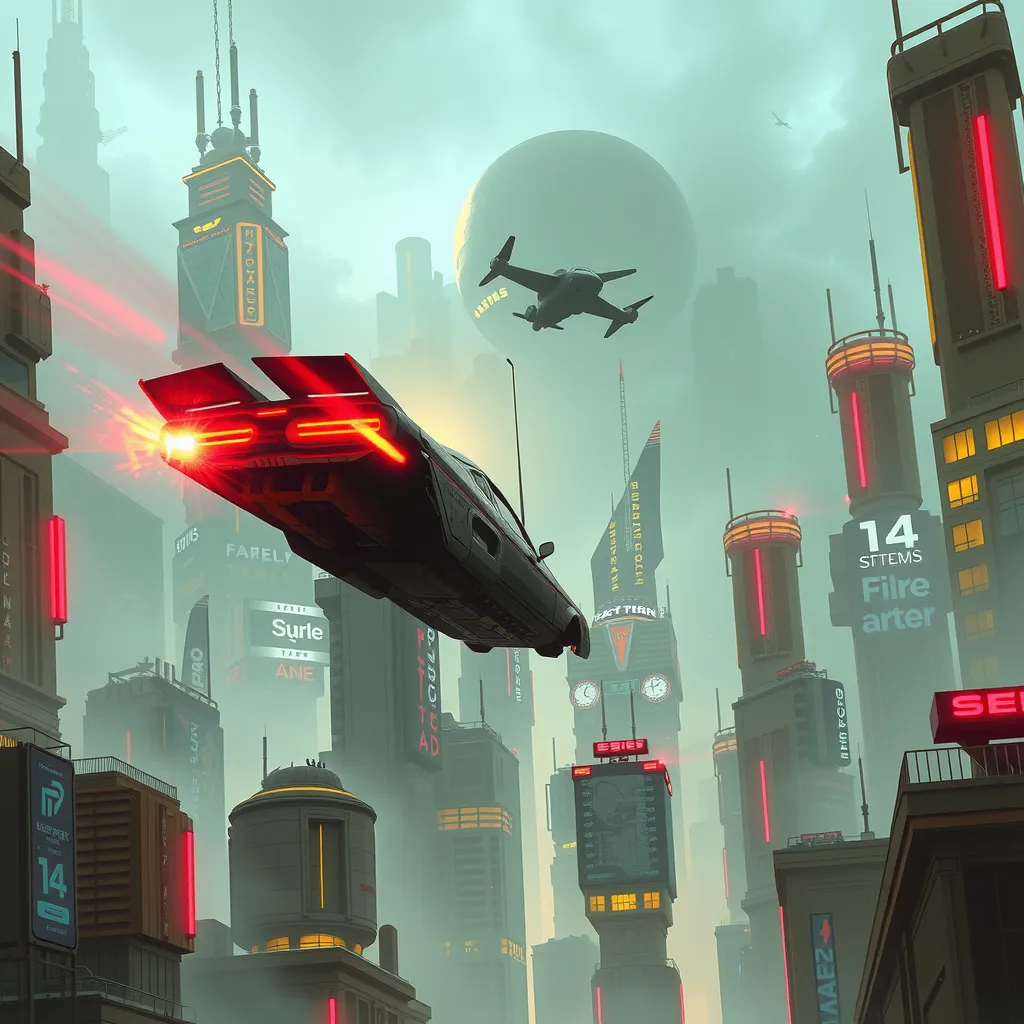
I usually use a guidance scale between 7 and 8. It gives a good mix of creativity and accuracy. Play around with these settings to find what works best for you.
Making Flux Faster
- Use the Schnell model for quick tests. It's faster but less detailed.
- Try fewer inference steps. For the
dev
model 30-50 is often good enough, and for theschnell
model 5 is good enough. - Use a GPU if you have one. It's much faster.
- For lots of images, use
--batch_size
. It's great for making many images at once.
Remember, faster isn't always better. Sometimes, making many quick images leads to better ideas than a few perfect ones. I've had some of my best creative breakthroughs by rapidly iterating with the Schnell model.
Different Ways to Use Flux
People use Flux in different ways. Some like to make lots of images automatically. Others prefer to carefully craft each image. I like a mix of both.
In my last project, I used Flux to generate hundreds of unique avatar images. The batch processing feature was a lifesaver. It saved me hours of work and the client was thrilled with the variety.
There are other tools like DALL-E and Midjourney. They're good too, but I prefer Flux because it works well with Python and gives me more control. It's all about finding the tool that fits your workflow best.
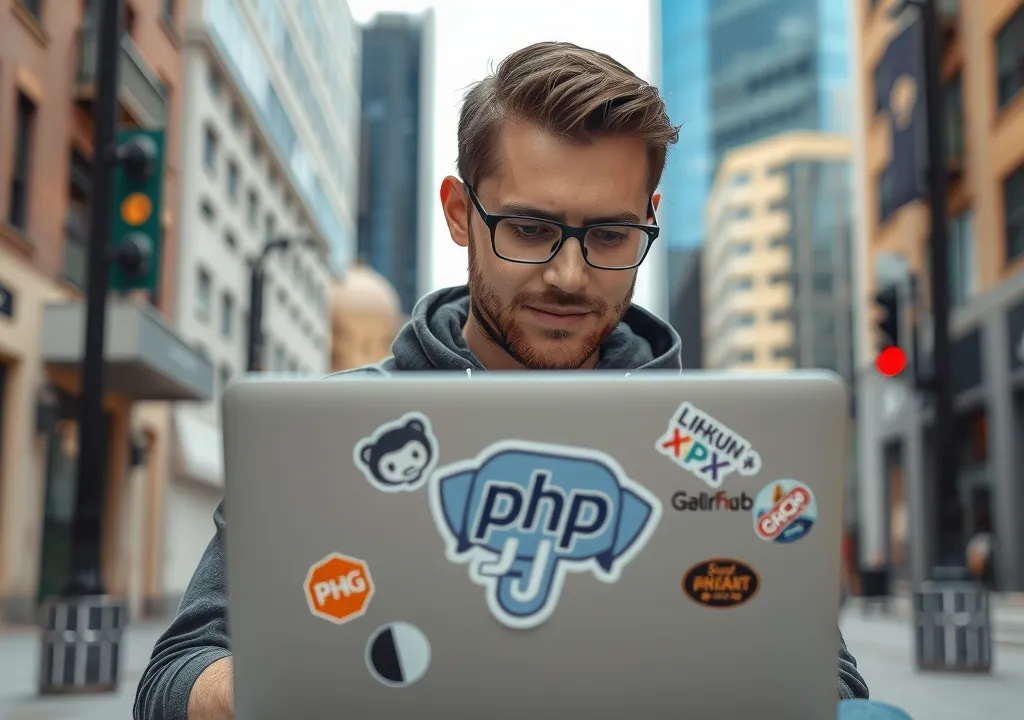
Wrapping Up
The key to mastering Flux is to experiment. Try new things. Push the limits. That's how you'll learn...
So what are you waiting for? Download the repository, fire up your Python environment and start creating with Flux. And hey, if you make something cool, share it with the community and let me know on x.com.
If you're looking to learn more about coding and fun side-projects, consider subscribing to my newsletter. I share weekly articles packed with developer tips and best practices.
- Flux Image Generation - Github Project
Happy coding!