PHP Football Data: A powerful PHP API package
I'm a big football enthusiast, and it's no secret that AS Roma is my favorite team. A few years back, while working on the AS Roma Club Norvegia website, I developed a package to fetch live and updated football data. Today, I'm excited to dust it off and share it with you: PHP Football Data. This package offers a clean and efficient way to interact with FootballData's API.
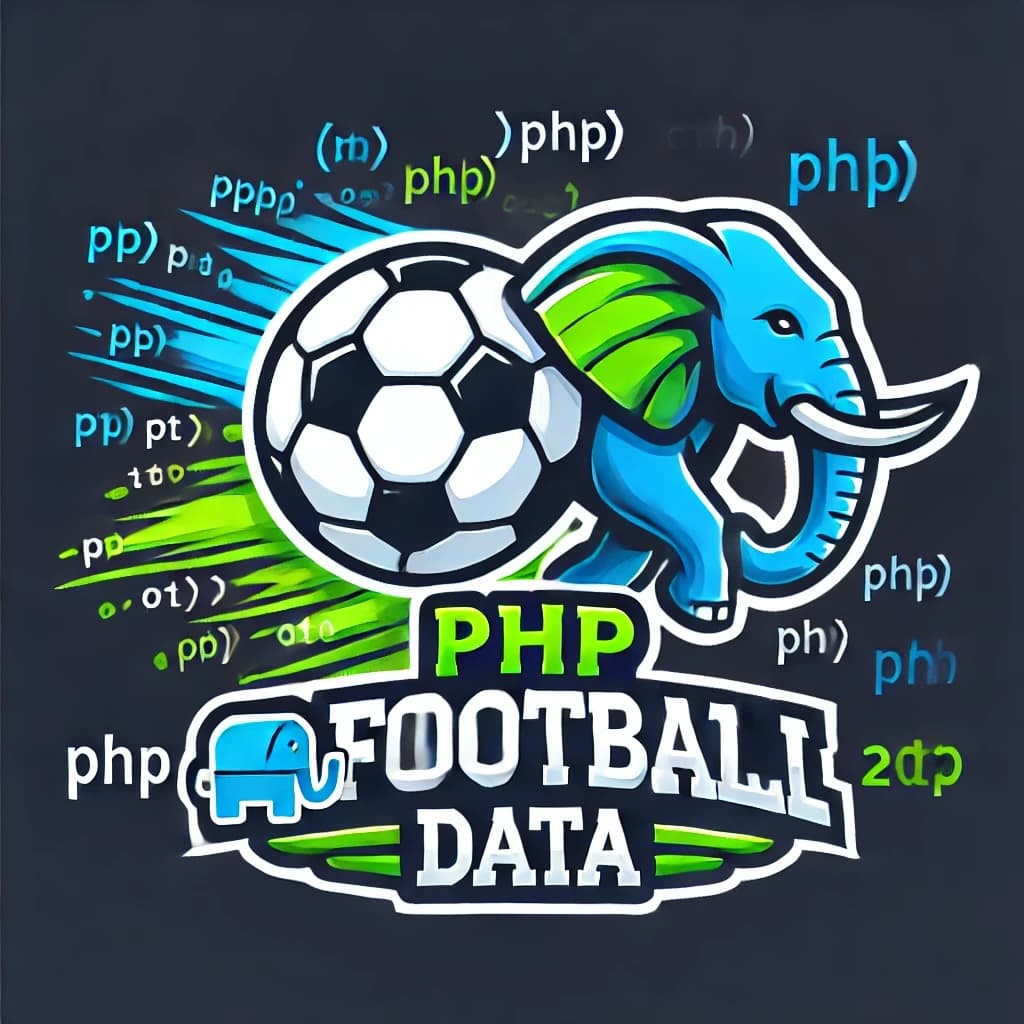
Key Features
-
PSR Compliance: The package adheres to PHP Standard Recommendations, ensuring compatibility and interoperability with a wide range of PHP projects.
-
Comprehensive API Coverage: Access data on areas, competitions, matches, teams, and individual players with ease.
-
Rate Limiting: Built-in support for managing API rate limits, crucial for staying within FootballData's usage restrictions.
-
Flexible Data Collections: Choose between eager loading or lazy collections for optimal performance based on your use case.
-
Laravel Integration: While the package works with any PHP project, it includes specific instructions for seamless Laravel integration.
Getting Started
Let's walk through the basics of setting up and using PHP Football Data in your project.
Installation
First, install the package via Composer:
composer require einar-hansen/php-football-data
Basic Usage
Here's a quick example of how you can start fetching football data:
use EinarHansen\FootballData\FootballDataService;
$service = new FootballDataService(apiToken: 'your-api-token-here');
// Fetch all competitions
$competitions = $service->competitions()->all();
// Find a specific team
$team = $service->teams()->find(66); // Manchester United FC
// Get matches for a competition
$matches = $service->competitions()->matches(2021); // Premier League matches
Advanced Features
The package offers more advanced features like pagination and rate limit tracking:
// Paginate through teams
$teamsPaginator = $service->teams()->paginate(limit: 50, page: 1);
// Move through pages
$nextPage = $teamsPaginator->nextPage();
$previousPage = $nextPage->previousPage();
// Track rate limits
$rateLimiter = new \EinarHansen\Http\RateLimit\Psr16RateLimitState(
cacheKey: 'football-data',
cache: $psr16Cache,
maxAttempts: 10,
decaySeconds: 60,
);
$service = new FootballDataService(
apiToken: 'your-api-token',
rateLimiterState: $rateLimiter,
);
Laravel Integration
For Laravel users, integrating the package is straightforward. Here's how you can set it up in your AppServiceProvider
:
use EinarHansen\FootballData\FootballDataService;
use EinarHansen\Http\RateLimit\Psr16RateLimitState;
use GuzzleHttp\Client;
class AppServiceProvider extends ServiceProvider
{
public function register()
{
$this->app->singleton(FootballDataService::class, function ($app) {
return new FootballDataService(
apiToken: $app['config']['services']['football-data']['api-token'],
client: new Client(),
rateLimiterState: new Psr16RateLimitState(
cacheKey: 'football-data',
cache: $app->make('cache.store'),
maxAttempts: 10,
decaySeconds: 60,
)
);
});
}
}
Wrapping Up
PHP Football Data aims to make working with football data in PHP projects as smooth and efficient as possible. Whether you're building a small hobby project or a large-scale application, this package provides the tools you need to easily integrate comprehensive football data.
I'm excited to see what the community builds with this package. If you have any questions, suggestions, or just want to share what you're working on, feel free to reach out or contribute to the GitHub repository.
Happy coding, and may your projects score big with PHP Football Data!