Simplifying File Compression in Laravel with Pinimize
Every now and then we find ourselves dealing with large files that need to be compressed for storage or transmission. While PHP provides built-in functions for compression, integrating these seamlessly into a Laravel application can be challenging and verbose. That's where Pinimize comes in - a powerful Laravel package that I've recently created, which simplifies file compression and decompression tasks.
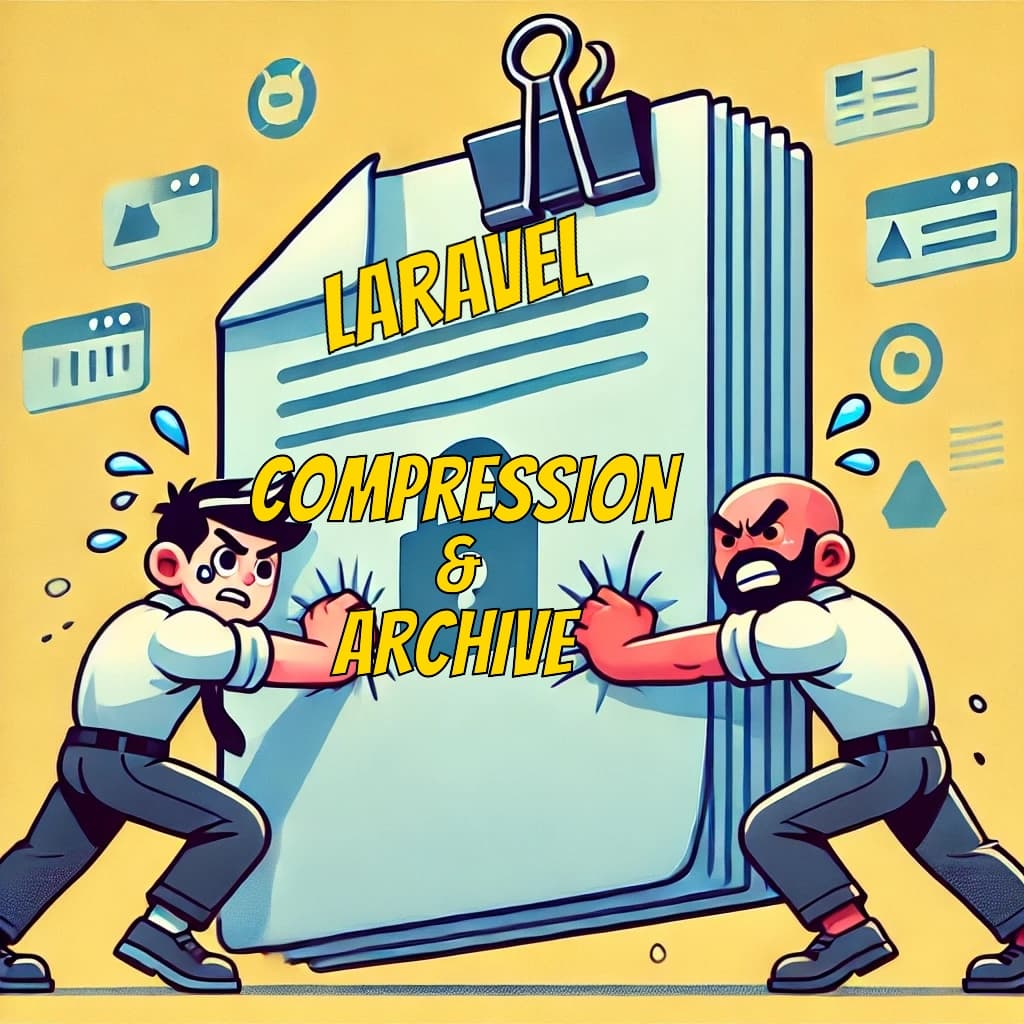
What is Pinimize?
Pinimize is a Laravel package that provides a clean and intuitive API for handling various compression and decompression tasks. It's designed to work seamlessly with Laravel's Storage system, making it a perfect fit for Laravel applications that need to handle file compression efficiently.
Key Features
-
Multiple Compression Methods: Pinimize supports both string and file compression, giving you flexibility in how you handle your data.
-
Stream Compression: For dealing with large files, Pinimize offers stream compression and decompression, allowing you to process data without loading everything into memory.
-
Laravel Storage Integration: The package fully integrates with Laravel's Storage system, making it easy to compress and decompress files on any configured disk.
-
Multiple Compression Algorithms: Currently supporting Gzip and Zlib, with more algorithms planned for future releases.
-
Extensible Architecture: You can easily add your own custom compression drivers if needed.
Getting Started with Pinimize
Let's walk through how to get started with Pinimize in your Laravel project. You can also find detailed installation instructions in the Pinimize GitHub repository.
Installation
First, install the package via Composer:
composer require pinimize/laravel-compression-and-archive
Configuration
After installation, publish the configuration file:
php artisan vendor:publish --provider="Pinimize\PinimizeServiceProvider" --tag="config"
This will create a config/pinimize.php
file where you can configure your compression settings.
Basic Usage
Pinimize extends Laravel's Str
and Storage
facades with convenient methods for compression and decompression. Let's look at some examples.
String Compression
use Illuminate\Support\Str;
$originalString = "This is a long string that will be compressed.";
$compressedString = Str::compress($originalString);
// To decompress
$decompressedString = Str::decompress($compressedString);
// Use a non-default driver
$decompressedString = Str::decompress($compressedString, 'zlib');
File Compression
use Illuminate\Support\Facades\Storage;
// Compress a file
$compressResult = Storage::compress('large_file.txt', 'compressed_file.gz');
// Compress a file, remove the initial file and specify a driver
$compressResult = Storage::compress('large_file.txt', 'compressed_file.gz', true, 'gzip');
// Decompress a file
$decompressResult = Storage::decompress('compressed_file.gz', 'decompressed_file.txt');
Working with Different Storage Disks
The package also provides you with the Compression
and Decompression
, they can be used for more advanced features. They integrate with both the local filesystem $options = ['disk' => null]
and Laravel's Storage system $options = ['disk' => 's3']
. This means you can easily compress and store files on any configured disk:
use Illuminate\Support\Facades\Compression;
use Illuminate\Support\Facades\Decompression;
// Compress and store on S3
Compression::put('compressed/output.gz', 'Content to compress', ['disk' => 's3']);
// Compress and store on Google Cloud Storage
Compression::put('gcs_output.gz', 'Content', ['disk' => 'gcs']);
// Compress and store on the local filesystem
Compression::put('/var/task/text.gz', 'Lorem ipsum dolor sit amet.', ['disk' => null]);
// Decompress and store on the local filesystem
$resource = fopen('/path/to/compressed_file.txt.gz', 'r');
Decompression::put('/path/to/decompressed_file.txt', $resource, ['disk' => null]);
Data types
One of the strengths of Pinimize's Compression
and Decompression
facades is their ability to work with various data types, making them versatile tools for different compression scenarios. Here's a quick rundown of what you can compress:
- Strings: Directly compress string content or file paths.
- PSR-7 StreamInterface: Perfect for working with HTTP messages.
- Laravel's File and UploadedFile objects: Seamlessly handle local and uploaded files.
- PHP resources: Work directly with file pointers.
This flexibility allows you to integrate Pinimize into your Laravel application regardless of how your data is structured. Whether you're dealing with user uploads, API responses, or locally stored files, Pinimize has you covered.
// Compress a string
Compression::put('output.gz', 'Content to compress');
// Compress an uploaded file
Compression::put('upload.gz', $request->file('document'));
// Compress from a resource
$resource = fopen('path/to/file.txt', 'r');
Compression::put('output.gz', $resource);
With Pinimize, you don't need to worry about converting your data to a specific format before compression. Just pass it to Pinimize, and it'll handle the rest!
Advanced Features
Downloading Compressed Files
One of the advanced features of Pinimize is its ability to create download responses for compressed files. This is particularly useful when you need to offer compressed files for download in your web application. Here's how you can use this feature:
use Illuminate\Support\Facades\Compression;
// Create a download response for a compressed file
return Compression::download('path/to/file.txt', 'downloaded_file.gz');
This method compresses the file on-the-fly and returns a response that will prompt the user to download the compressed file. You can also use it to return decompressed files for download:
use Illuminate\Support\Facades\Decompression;
// Create a download response for a decompressed file
return Decompression::download('path/to/file.txt.gz', 'decompressed_file.txt');
This method decompresses the file before creating the download response, allowing users to download the decompressed version of the file.
Custom Drivers
For those with specific compression needs, Pinimize allows you to create and register custom compression drivers:
use Illuminate\Support\Facades\Compression;
public function boot()
{
Compression::extend('custom', function ($app) {
return new CustomCompressionDriver($app['config']['compression.custom']);
});
}
Why Use Pinimize?
-
Simplicity: Pinimize provides a clean, Laravel-style API for compression tasks, making your code more readable and maintainable.
-
Flexibility: With support for multiple compression algorithms and the ability to work with strings, files, and streams, Pinimize can handle a wide range of compression scenarios.
-
Performance: By offering stream compression and decompression, Pinimize allows you to work with large files efficiently, without exhausting your server's memory.
-
Integration: The seamless integration with Laravel's Storage system means you can easily compress and decompress files on any storage disk your application uses.
Wrapping Up
Pinimize is a powerful tool that simplifies file compression tasks in Laravel applications. Whether you're dealing with large file uploads, need to archive data, or are looking to optimize your storage usage, Pinimize provides an elegant solution that integrates smoothly with Laravel's existing features.
By leveraging Pinimize in your Laravel projects, you can write cleaner, more efficient code for handling file compression, ultimately leading to better performing and more maintainable applications.
Give Pinimize a try in your next Laravel project and experience the difference it can make in handling your file compression needs!
Links:
Happy coding!